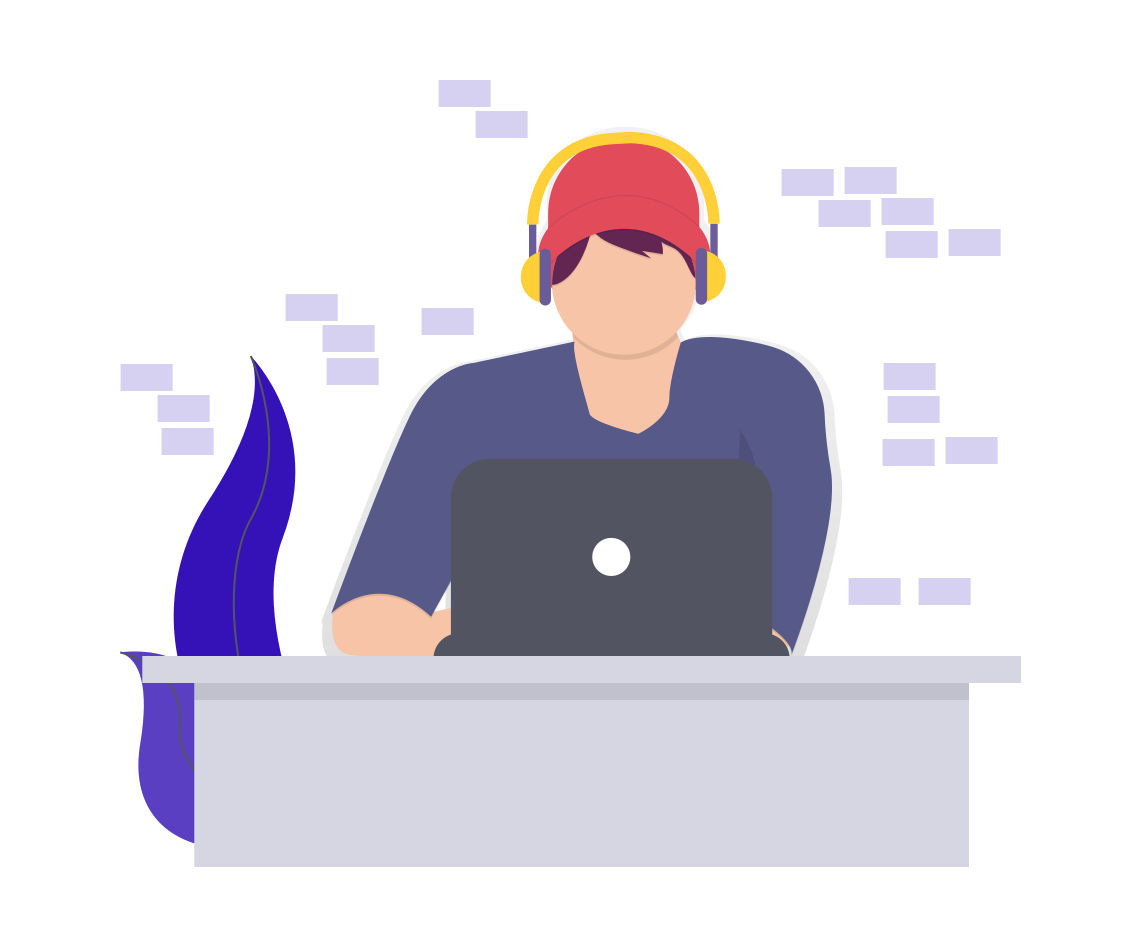
Unity C# Refresher
Why C#? When it comes to Unity scripting, an early question when making a new game is which language to choose, because Unity offers a choice. The official choices are C# or JavaScript. However, there's a debate about whether JavaScript should more properly be named "JavaScript" or "UnityScript" due to the Unity-specific adaptations made to the language.
This point is not our concern here. The question is which language should be chosen for your project. Now, it initially seems that as we have a choice, we can actually choose all two languages and write some script filesin one language and other script files in another language, thus effectively mixing up the languages. This is, of course, technically possible. Unity won't stop you from doing this. However, it's a "bad" practice because it typically leads to confusion as well as compilation conflicts; it's like trying to calculate distances in miles and kilometers at the same time.
C#. Why? First, it's not because C# is "better" than the others. There is no absolute "better" or "worse" in my view. Each and every language has its own merits and uses, and all the Unity languages are equally serviceable for making games.
The main reason is that C# is, perhaps, the most widely used and supported Unity language, because it connects most readily to the existing knowledge that most developers already have when they approach Unity. Most Unity tutorials are written with C# in mind, as it has a strong presence in other fields of application development. C# is historically tied to the .NET framework, which is also used in Unity (known as Mono there), and C# most closely resembles C++, which generally has a strong presence in game development. Further, by learning C#, you're more likely to find that your skill set aligns with the current demand for Unity programmers in the contemporary games industry.
Variables
Variables often correspond to the letters used in algebra and stand in for numerical quantities, such as X, Y, and Z and a, b, and c. If you need to keep track of information, such as the player name, score, position, orientation, ammo, health, and a multitude of other types of quantifiable data.
A variable represents a single unit of information. This means that multiple variables are needed to hold multiple units, one variable for each.
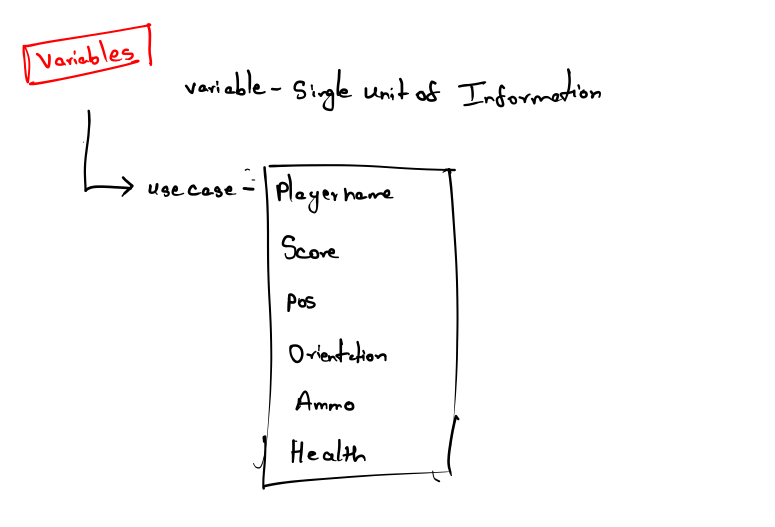
public class MyNewScript : MonoBehaviour
{
public string PlayerName = "";
public int PlayerHealth = 100;
public Vector3 Position = Vector3.zero;
}
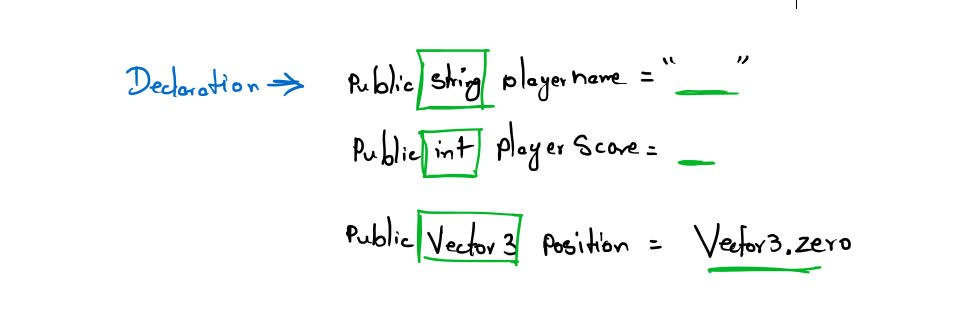
Usually, variables can be either public or private protected.The public variables will be accessible and editable in Unity's Object Inspector, and they can also be accessed by other classes.
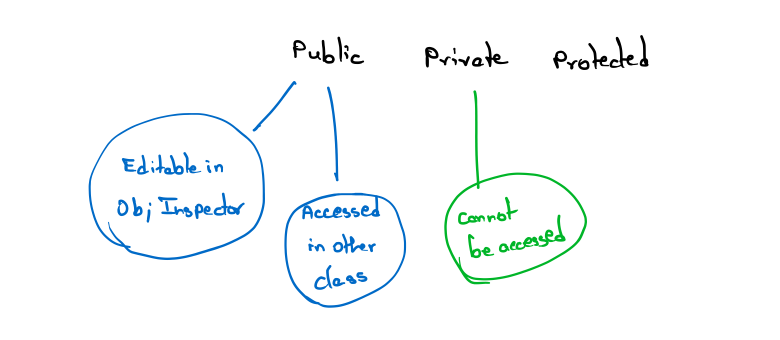
Conditional statements
Variables change in potentially many different circumstances: when the player changes their position, when enemies are destroyed, when the level changes, and so on. Consequently, you'll frequently need to check the value of a variable to branch the execution of your scripts that perform different sets of actions, depending on the value.
if statement
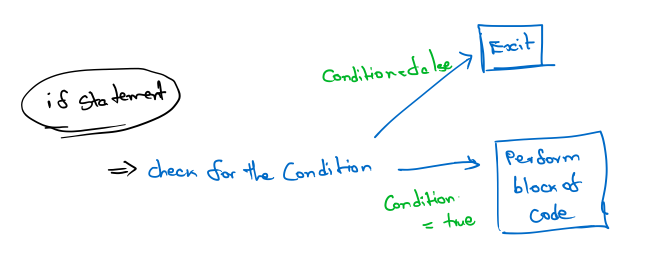
if(PlayerHealth == 100)
{
Debug.Log ("Player has full health");
}
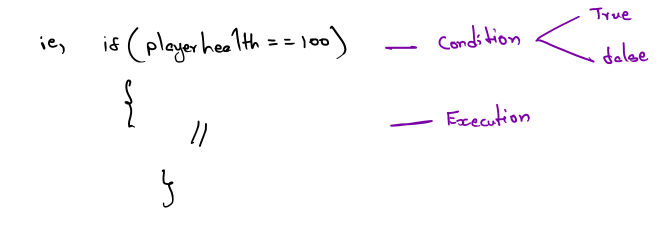
switch statement
The switch statement, in contrast, lets you check a variable for multiple possible conditions or states, and then lets you branch the program in one of many possible directions, not just one or two as is the case with if statements.
public class MyScriptFile : MonoBehaviour{
public enum EnemyState {CHASE, FLEE, FIGHT, HIDE};
public EnemyState ActiveState = EnemyState.CHASE;
void Update()
{
switch(ActiveState)
{
case EnemyState.FIGHT:
{
Debug.Log ("Entered fight state");
}
break;
}
}
}
An enum is a special structure used to store a range of potential values for one or more other variables. It's not a variable itself per se, but a way of specifying the limits of values that a variable might have.
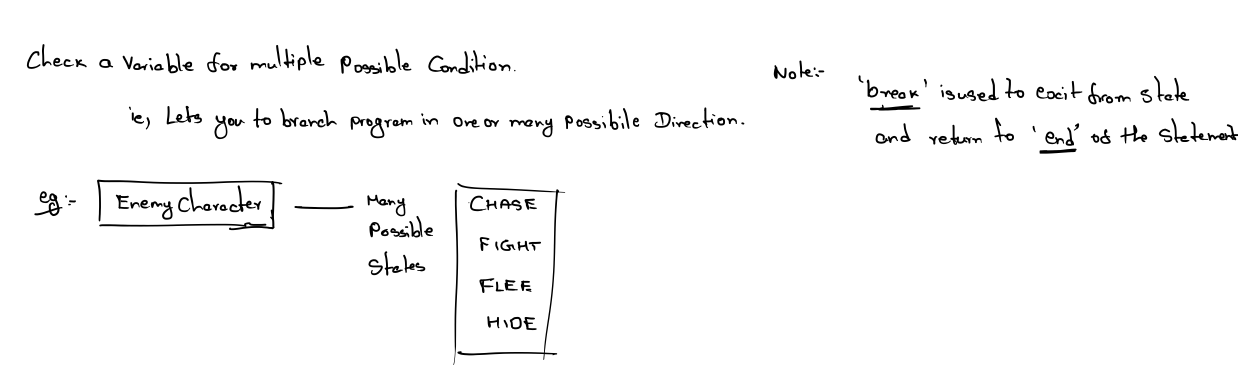
Arrays
Lists and sequences are everywhere in games.Each item in the array is, essentially, a unit of information that has the potential to change during gameplay, and so a variable is suitable to store each item.
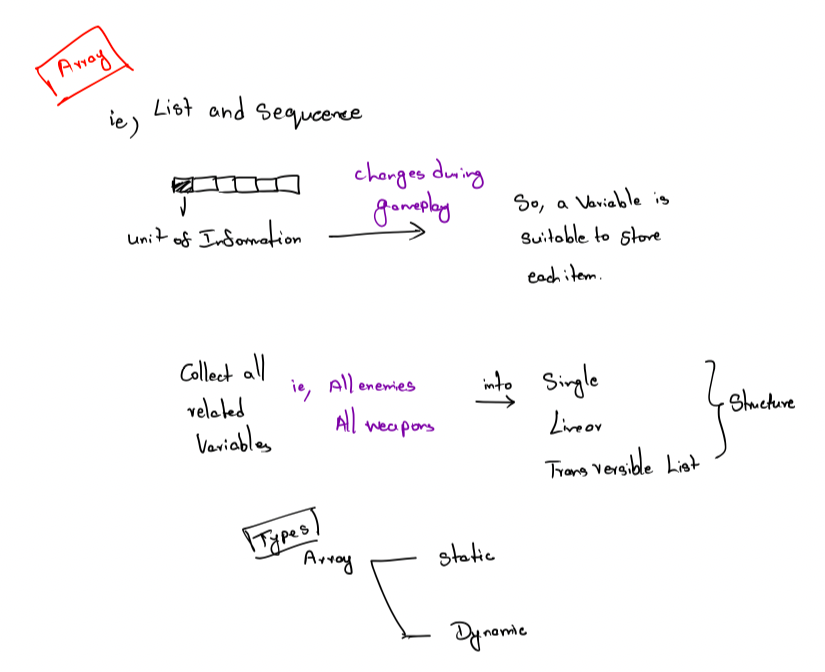
In C#, there are two kinds of arrays:static and dynamic .
Static arrays might hold a fixed and maximum number of possible entries in memory, decided in advance, and this capacity remains unchanged throughout program execution, even if you only need to store fewer items than the capacity. This means some slots or entries could be wasted.
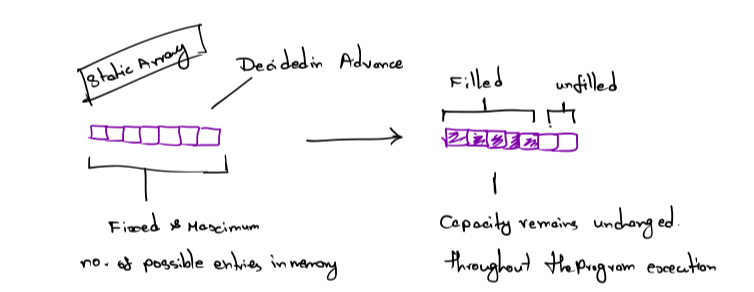
Dynamic arrays might grow and shrink in capacity, on demand, to accommodate exactly the number of items required. Static arrays typically perform better and faster, but dynamic arrays feel cleaner and avoid memory wastage.
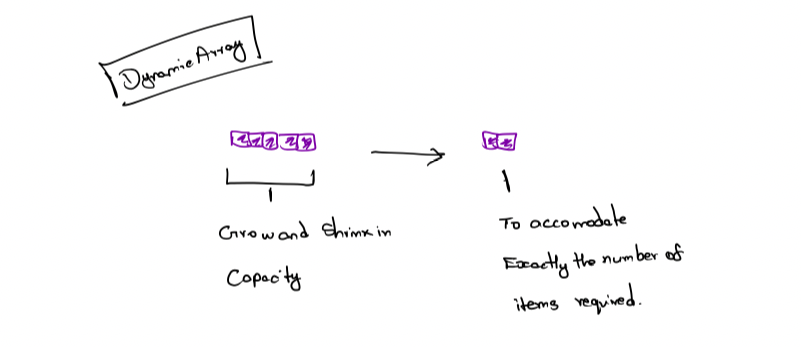
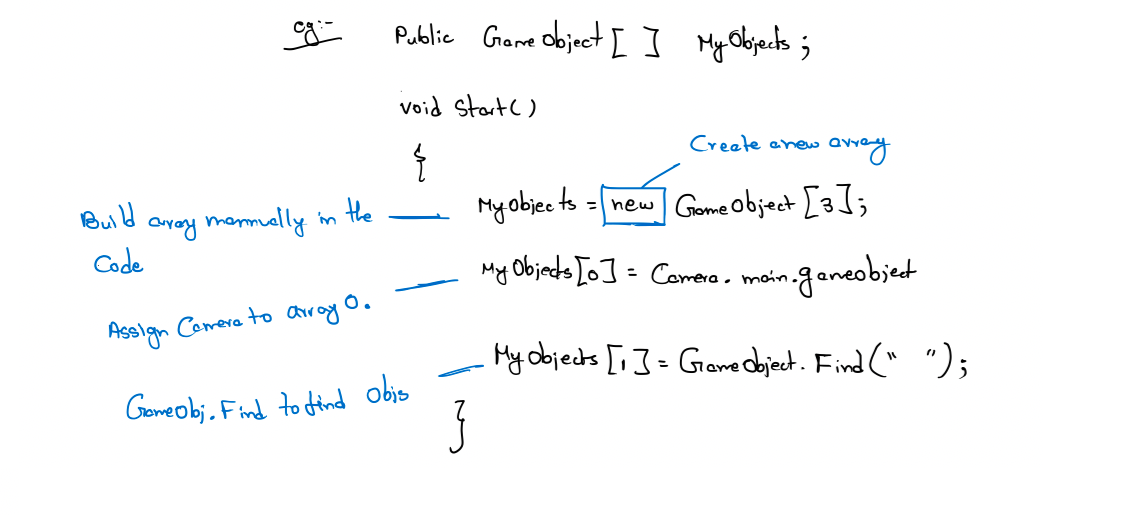
LOOPS
Loops are one of the most powerful tools in programming. Imagine a game where the entire level can be nuked. When this happens, you'll want to destroy almost everything in the scene. Now, you can do this by deleting each and every object individually in code, one line at a time. If you did this, then a small scene with only a few objects would take just a few lines of code, and this wouldn't be problematic. However, for larger scenes with potentially hundreds of objects, you'd have to write a lot of code, and this code would need to be changed if you altered the contents of the scene. This would be tedious. Loops can simplify the process to just a few lines, regardless of scene complexity or object number.
Foreach loop
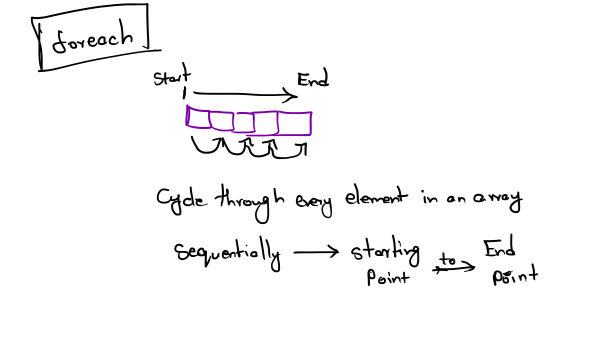
Foreach, you can cycle through every element in an array, sequentially from start to end, processing each item as required.
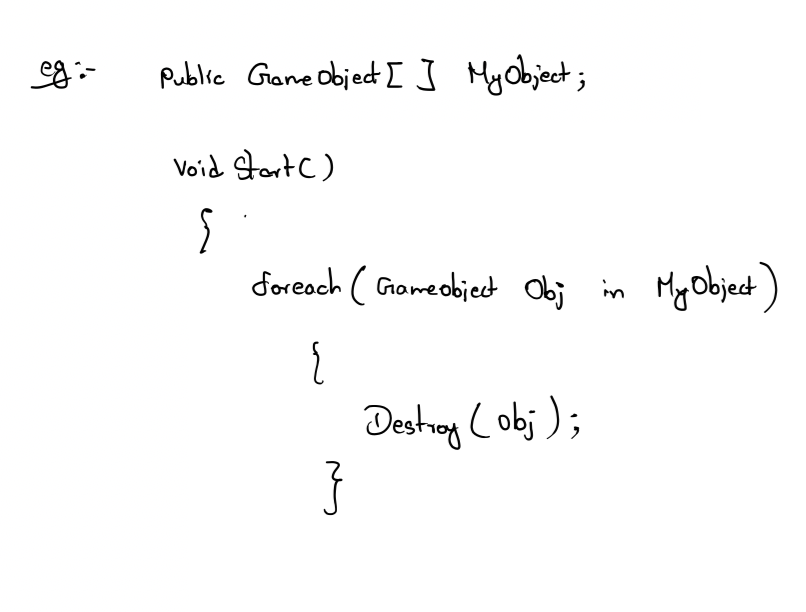
The foreach loop repeats the code block {} , once for each element in the array MyObjects. Each pass or cycle in the loop is known as an iteration. The loop depends on array size; this means that larger arrays require more iterations and more processing time. The loop also features a local variable obj. This is declared in the foreach statement. This variable stands in for the selected or active element in the array as the loop passes each iteration, so obj represents the first element in the loop on the first iteration, the second element on the second iteration, and so on.
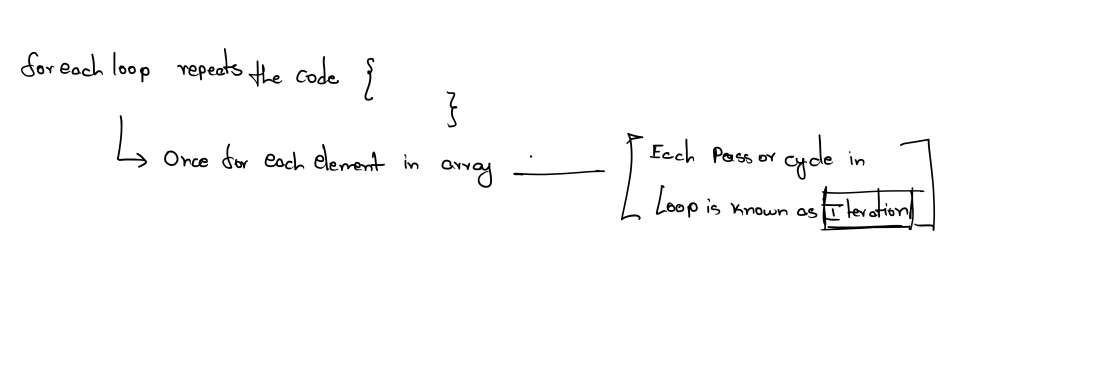