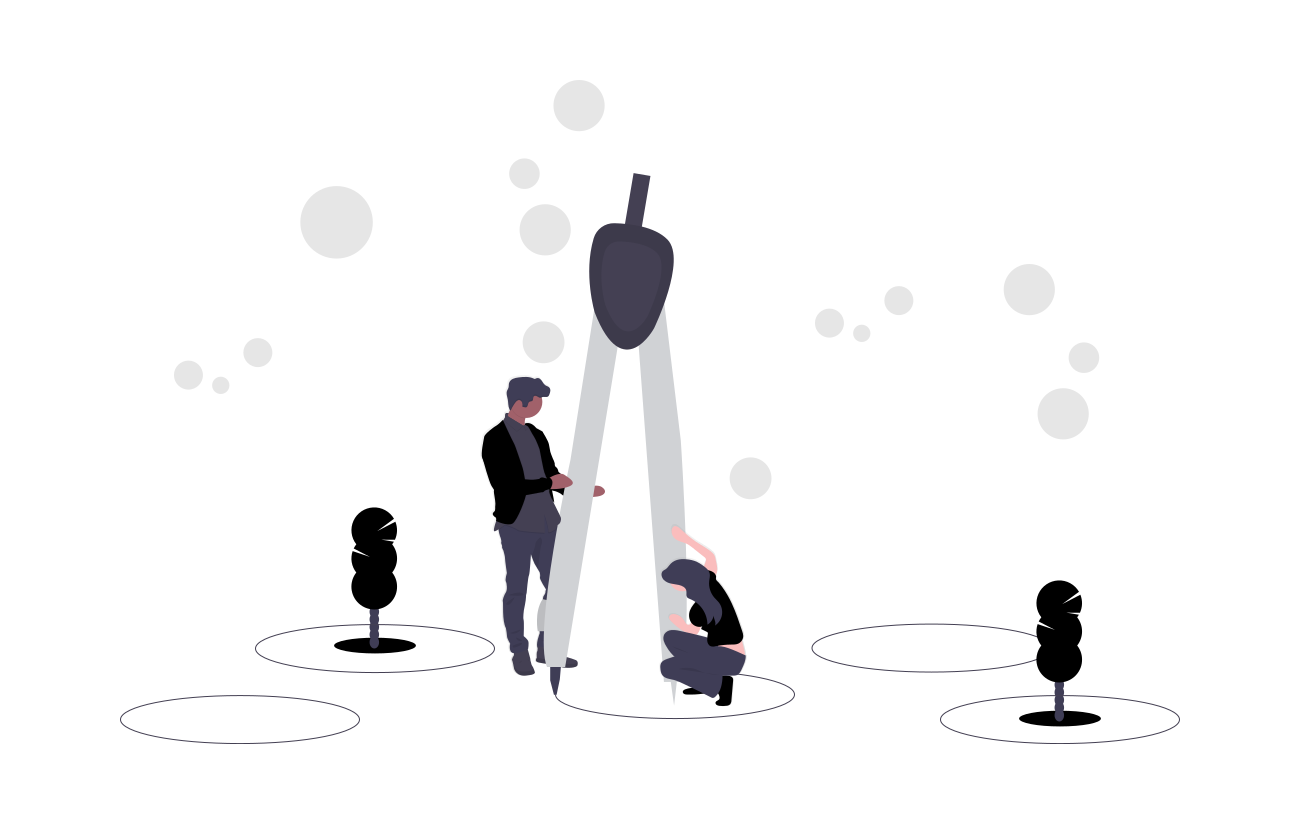
Shape Editor Tool
Why Editor Tools? The Unity Editor is (undeniably) an awesome engine for building games, but often gets this close to actually fitting your needs. At times, you probably find yourself wishing for a “do what I mean” button. Although Unity isn’t quite capable of interpreting brainwaves through some mystical button, there ways to extend this tool to make it more useful and user-friendly.
Unity is an amazing game engine that’s been on top of the game for a little more than a decade.It’s welcoming enough that students and indie game developers use it for learning the basics and working on small projects, yet it’s packed with enough features that full-fledged game studios use it too.
If you have been working with Unity for any amount of time or have worked on large Unity projects, you’ve no doubt realized how cumbersome it is when you have to perform repetitive tasks.Something as simple as calling PlayerPrefs.DeleteAll() requires you to run the scene, which could take a couple of seconds when you’re working on a large scene that takes a while to load all the assets.Even when you look for a 3rd-party tool in the Asset Store, either you can’t find something that’s within your budget, or the solution for your particular use case doesn’t exist.It’s for these specific reasons, along with many others, that Unity comes with an Editor Scripting API. With the Unity Editor Scripting API, users can extend the capabilities of the editor by building tools and utilities as per their requirements.
Simply put, you can write an Editor Script that uses methods from the UnityEditor namespace to create or modify custom functionalities to the Unity editor according to your requirements.There are many advantages to knowing the basics of editor scripting:
- As the size and complexity of your project increases.
- you would want a way to manage the huge amount of information that’s displayed in the Inspector.
You might want to execute a part of the code without having to run a scene every few minutes.You may want to give the designers a way to add or modify the assets that are manually integrated within the code.In all these cases, having basic knowledge of editor scripting can save you and your team a lot of time and money!The point is, as a developer, it’s good practice to know all of the various tools at your disposal that can help improve your workflow. Being able to modify an action so that the time spent doing it reduces from 20 seconds to 5 seconds can save you many hours during the entire development process.
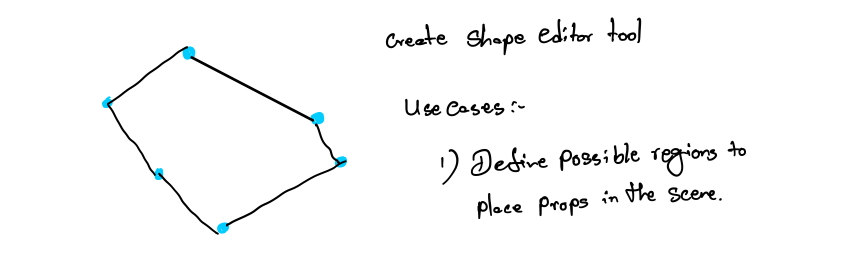
Functionality needed for the Unity Custom Editor - Shape Creator
The Basic Math behind the scenes are -
- Project a Ray in a Direction.
- Pass the Ray for a Particular Distance.
- Find the Draw Point.
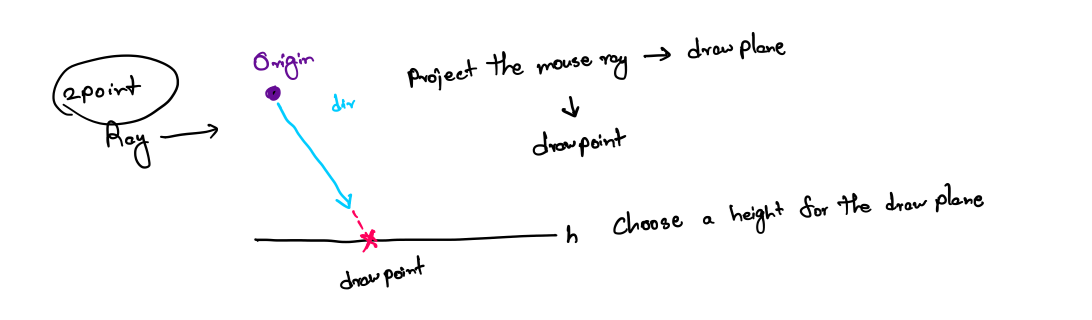
From the above diagram we can figure out a equation -
Origin + direction * Distance = DrawPoint(P).
Rewrite the Equation using the y -component of the values.
Since Point P -y axis represents the height of the plane P.y = h
Use Unity Editor and Inherit the Editor to use its functions.
The On Screen GUI method is called when there is a input in the scene.
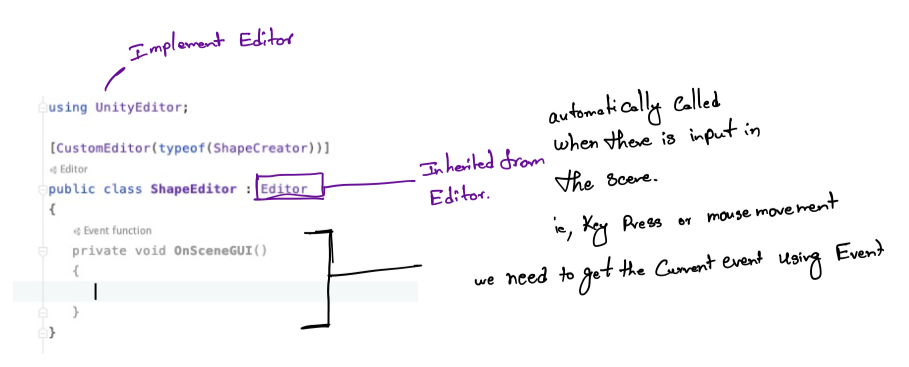
Now, we need to pass the ray from the mouseposition to the plane and subitute the above equation to find the point. Next,if we click on the button then find the point where the mouse is clicked.
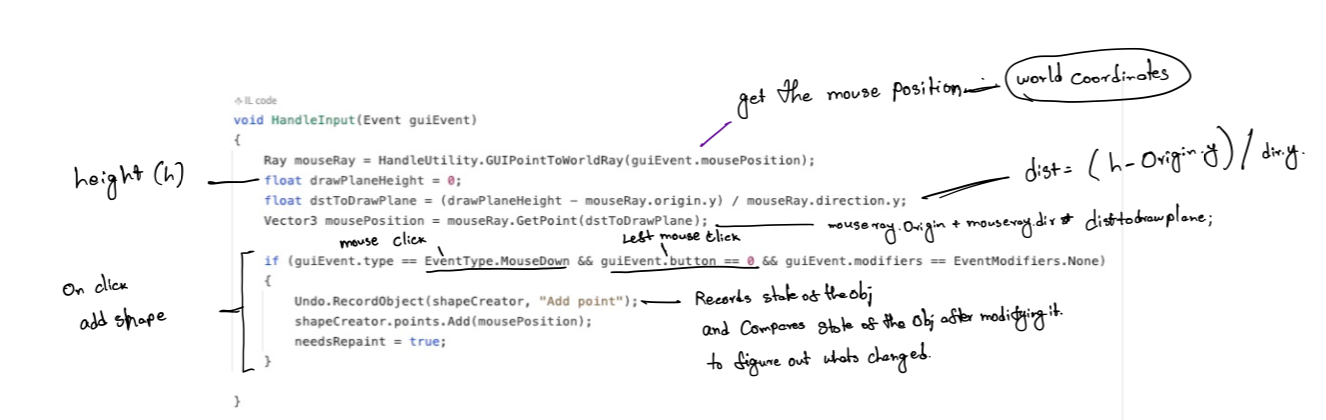
void HandleInput(Event guiEvent)
{
Ray mouseRay = HandleUtility.GUIPointToWorldRay(guiEvent.mousePosition);
float drawHeightPlane = 0;
float dstToDrawPlane = (drawHeightPlane - mouseRay.origin.y) / mouseRay.direction.y;
Vector3 mousePosition = mouseRay.GetPoint(dstToDrawPlane);
if (guiEvent.type == EventType.MouseDown && guiEvent.button ==0)
{
Undo.RecordObject(_shapeCreator,"Add Point");
_shapeCreator.points.Add(mousePosition);
Debug.Log( "Add"+mousePosition);
_needsRepaint = true;
}
}
In the Above video- you can see that it doesnt create a disc as soon i clicked on the scene, because the scene need to be repainted everytime when i clicked on the scene.
if (_needsRepaint)
{
HandleUtility.Repaint();
}
Since i click the Scene the selected item gets off, to resolve this issue just add a HandleUtility.AddDefaultController to the script.
Finally, Draw the SolidDisc from the points and add the points by the dotted Lines.